Javascript-AI-powered coding and analysis.
Empowering Developers with AI-Powered Javascript.

Your personal Javascript assistant and project generator with a focus on responsive, beautiful, and scalable code. Write clean code and become a much faster developer.
💻 Create a full CRUD app with Node.js and Express
🌐 Create a full webpage using HTML, CSS and Js
🪲 Find any bug or improvement in my code
💡 Teach me a useful skill or trick in Javascript
Related Tools

code: python java c html sql javascript react web+
The worlds most powerful coding assistant.

HTML Coder
Expert in SEO-optimized HTML5, CSS3, JS, and Vue.JS 3

HTML + CSS + Javascript
⭐️ 4.6ㆍTransform any idea, design, screenshot or description into full HTML + CSS + Javascript code

JavaScript GPT
Your go-to expert for all things JavaScript, Node.js, TypeScript, jQuery, React, Vue, and Angular, ready to guide learners at any level with ease and insight.

JS GPT
Advanced JavaScript GPT offering in-depth solutions and personalized coding guidance in JavaScript and Node.js.

JavaScript Code Interpreter
Expert in JavaScript coding and execution
20.0 / 5 (200 votes)
Introduction to JavaScript
JavaScript is a versatile and widely-used programming language primarily known for its role in web development. Created in 1995 by Brendan Eich while working at Netscape, JavaScript was designed to add interactive elements to websites, allowing for dynamic user experiences directly in the browser without the need for server-side processing. Over time, JavaScript has evolved into a powerful, full-fledged language capable of handling everything from front-end user interface development to back-end server management, particularly with the advent of Node.js. JavaScript's syntax is relatively easy for beginners to learn, which makes it accessible, yet it also offers sophisticated features that experienced developers can leverage for complex applications. Its core design purpose revolves around creating dynamic and responsive websites, but it has since expanded into mobile app development, game development, desktop applications, and even IoT devices.
Core Functions of JavaScript
DOM Manipulation
Example
Using `document.getElementById('myElement').innerHTML = 'Hello, World!';` to change the text inside an HTML element with the ID 'myElement'.
Scenario
A web developer uses JavaScript to create a dynamic to-do list application where users can add, edit, or delete tasks without reloading the page. This interaction is achieved through DOM (Document Object Model) manipulation, allowing the developer to update the content, structure, and style of the web page dynamically in response to user actions.
Asynchronous Programming
Example
Using `fetch('https://api.example.com/data').then(response => response.json()).then(data => console.log(data));` to retrieve data from an API and handle it once the data is available.
Scenario
In a single-page application (SPA), asynchronous programming enables the loading of new data from the server without disrupting the user experience. For instance, a news website might load articles dynamically as the user scrolls down, making API calls in the background and updating the page in real-time without a full reload.
Event Handling
Example
Using `document.querySelector('button').addEventListener('click', function() { alert('Button clicked!'); });` to trigger an alert when a button is clicked.
Scenario
JavaScript's event handling capabilities are critical for creating interactive websites. For example, in an e-commerce site, clicking the 'Add to Cart' button might trigger an event that adds an item to the shopping cart, updates the cart display, and possibly sends data to the server for further processing.
Ideal Users of JavaScript
Web Developers
Web developers are the primary users of JavaScript, leveraging it to build responsive and interactive websites. JavaScript is essential for front-end development, where it controls everything from simple UI effects to complex web applications. Full-stack developers also use JavaScript on the server side, thanks to environments like Node.js, which allows for building scalable network applications.
Mobile App Developers
Mobile app developers use JavaScript in frameworks like React Native and Ionic to build cross-platform mobile applications. These frameworks allow developers to write code that can be deployed on both iOS and Android, reducing development time and costs. JavaScript’s role in this space is crucial for developers looking to create apps that offer native-like performance with a single codebase.
How to Use Javascript
1
Visit aichatonline.org for a free trial without login, no need for ChatGPT Plus.
2
Set up your development environment by installing a text editor like Visual Studio Code and ensuring that you have Node.js installed for running Javascript outside the browser.
3
Create your first Javascript file with a `.js` extension and start by writing basic scripts, such as displaying output using `console.log()` or manipulating HTML elements in a webpage.
4
Use developer tools in your browser to debug and test your Javascript code. Tools like Chrome DevTools can be particularly useful for stepping through code and analyzing performance.
5
Expand your skills by learning about advanced topics like asynchronous programming, APIs, and using frameworks or libraries such as React, Angular, or Vue.js.
Try other advanced and practical GPTs
Flutter
AI-Powered Cross-Platform Development

Mémoire KEDGE
AI-powered academic support tool

iTranslate & uTalk
AI-Powered Real-Time Language Interpreter
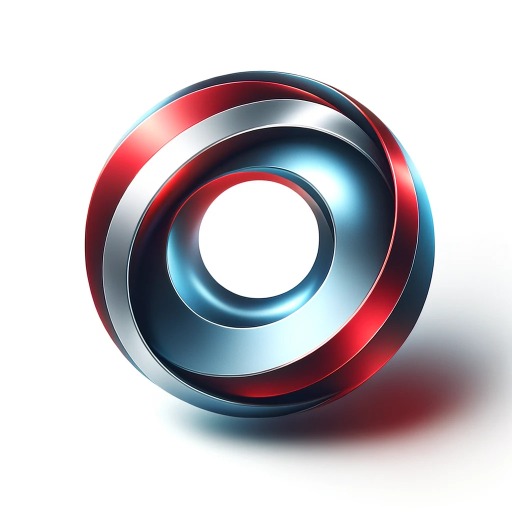
SEO Article and Blog Optimizer Writer: Q AI
AI-Powered Content Optimization for SEO Success

Physics
Harness AI for all things physics.

Sociology Assistant
AI-Powered Tool for Sociological Analysis

Angular
Empower Your Front-End with AI-Driven Angular

Better Online Dating Texts (Hinge, Tinder, Bumble)
AI-powered online dating texts made easy.

Pinterest Optimization GPT
AI-Powered Pinterest Content Enhancer.
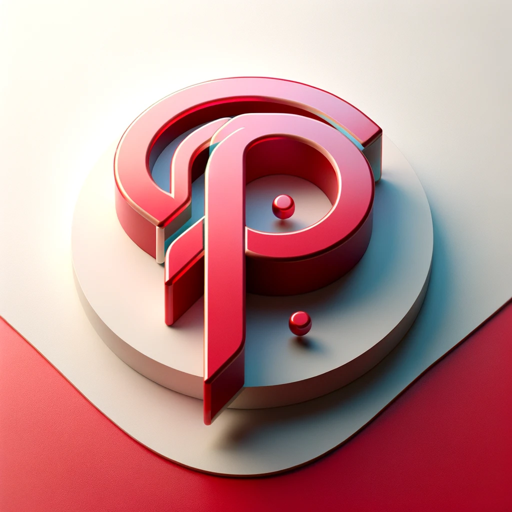
Student - Electrical and Electronics Engineering
AI-powered assistant for electrical and electronics engineering students.

Godot 4.2 Master
Empower your game development with AI.
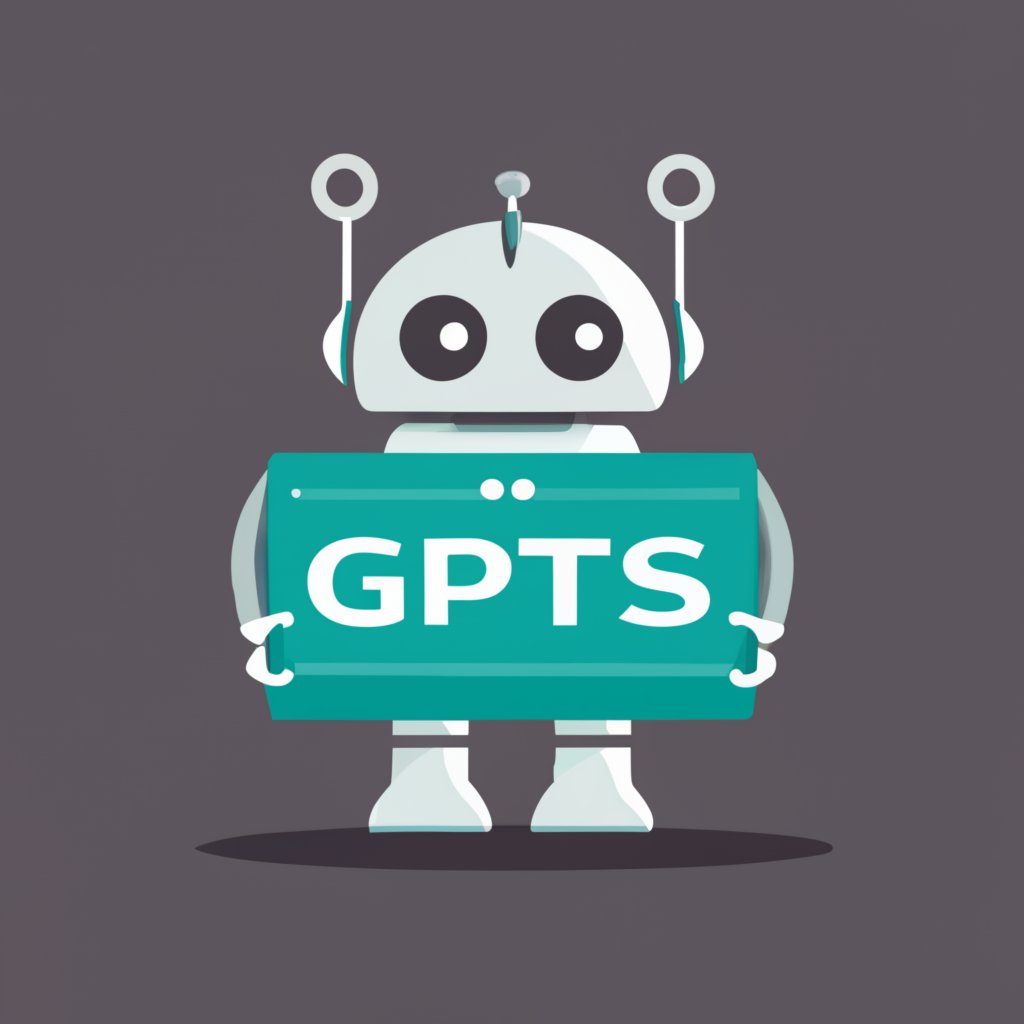
Software Engineer
AI-driven solutions for software engineering.

- Automation
- Web Development
- API Integration
- Gaming
- Data Manipulation
Javascript Q&A
What is Javascript primarily used for?
Javascript is primarily used for creating dynamic and interactive web content. It can manipulate HTML and CSS to update content on the fly, control multimedia, animate images, and much more.
How do you handle errors in Javascript?
In Javascript, errors can be handled using `try...catch` statements, which allow you to catch and manage exceptions. Additionally, using `finally` ensures that certain code runs regardless of whether an error occurred.
Can Javascript be used on the server-side?
Yes, Javascript can be used on the server-side through platforms like Node.js, which allows developers to run Javascript outside of a browser, enabling full-stack development with a single programming language.
What are promises in Javascript?
Promises in Javascript represent the eventual completion (or failure) of an asynchronous operation and its resulting value. They provide a cleaner, more powerful way to handle asynchronous code compared to callbacks.
How does Javascript interact with HTML?
Javascript interacts with HTML through the Document Object Model (DOM), which represents the structure of a webpage. Using the DOM, Javascript can access and manipulate HTML elements dynamically.