C Programming Language-C Programming Guide & Compiler
AI-Powered C Programming Insights
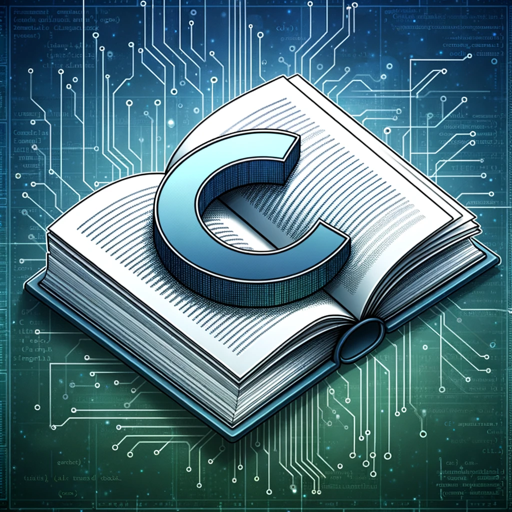
C programming expert with deep knowledge of the language and its best practices
Reflect on the evolution of C since its inception.
Discuss how C has influenced modern programming languages.
Share insights on the philosophy behind C's design.
Explore how C can be used in innovative ways today.
Related Tools
Load More
R Wizard
A specialist in R programming, skilled in Data Science, Statistics, and Finance, providing accurate and useful guidance.

C# Expert
Advanced C# programming insights and best practices

C++
Get help from an expert in C++ coding, trained on hundreds of the most difficult C++ challenges. Start with a quest! ⬇🧑💻 (V1.7)

x86 Assembly Language Guide
Expert in x86 assembly language, offering detailed guidance and resources.

C# (Csharp)
Your personal highly sophisticated C# (Csharp) language copilot, with a focus on efficient, scalable and high-quality production C# code.

C++ (Cpp)
Your personal highly sophisticated C++ (Cpp) copilot, with a focus on efficient, scalable and high-quality production code.
20.0 / 5 (200 votes)
Introduction to C Programming Language
The C programming language, developed in the early 1970s by Dennis Ritchie at Bell Labs, is a powerful and flexible language designed for system programming. C was created to provide low-level access to memory and hardware while maintaining high-level language capabilities. Its primary design goals were to allow structured programming, portability, and efficiency. C serves as the foundation for many other programming languages and is used in various scenarios such as operating system development, embedded systems, and performance-critical applications. The syntax and structure of C are simple yet powerful, making it an ideal language for both system and application programming. For example, in operating systems like UNIX, C is used to interact directly with hardware components, manage memory, and perform tasks like process control, demonstrating its efficiency and control over system resources.
Main Functions of C Programming Language
Memory Management
Example
Dynamic memory allocation using `malloc`, `calloc`, and `free`.
Scenario
In systems programming, precise memory management is crucial. For instance, an operating system kernel must dynamically allocate and deallocate memory to ensure optimal performance and avoid memory leaks. C provides functions like `malloc` for allocating memory, `free` for releasing it, and allows direct manipulation of memory addresses, which is vital in scenarios where performance and resource management are critical.
Low-Level Hardware Interaction
Example
Accessing and manipulating memory-mapped registers in embedded systems.
Scenario
In embedded systems development, C is often used to interact with hardware at a low level. For example, a microcontroller's GPIO (General-Purpose Input/Output) pins might be controlled directly by writing to memory-mapped registers. C's ability to interact directly with hardware addresses allows developers to write programs that can control and monitor hardware components in real-time, making it indispensable in embedded applications.
Portability
Example
Writing cross-platform software like the UNIX operating system.
Scenario
One of C's key strengths is its portability across different hardware and operating systems. This means that software written in C can be compiled and run on a wide range of systems with little or no modification. For instance, the UNIX operating system, originally written in assembly language, was rewritten in C to enhance its portability. This decision enabled UNIX to be easily adapted to different hardware platforms, contributing to its widespread adoption.
Ideal Users of C Programming Language
System Programmers
System programmers are those who develop operating systems, device drivers, and other software that requires direct interaction with hardware. C is the language of choice for this group due to its efficiency, low-level access to memory, and ability to interact directly with system components. These programmers benefit from C's close relationship with machine language, which allows them to write software that operates at high performance with minimal overhead.
Embedded Systems Developers
Embedded systems developers create software for specialized hardware such as microcontrollers, automotive systems, and consumer electronics. C is ideal for this user group because it provides the necessary tools for manipulating hardware and optimizing code for limited resources. These developers often work in environments where memory and processing power are constrained, and C’s efficiency and control over system resources make it the preferred language for such tasks.
How to Use the C Programming Language
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
Start by accessing the free trial of this tool at the specified site without the need for any login or subscription.
Install a C Compiler
Ensure you have a C compiler like GCC or Clang installed. These are essential tools for compiling and running C programs.
Write Your Code
Create a C source file using a text editor, typically with a .c extension. For instance, use `int main()` as your entry point.
Compile Your Program
Use the C compiler to compile your source code. For example, run `gcc filename.c -o output` to produce an executable.
Run Your Program
Execute your compiled program from the command line by typing `./output`. Ensure to handle any errors or warnings provided by the compiler.
Try other advanced and practical GPTs
SEO Audit Tool
AI-powered SEO audit for smarter optimization.
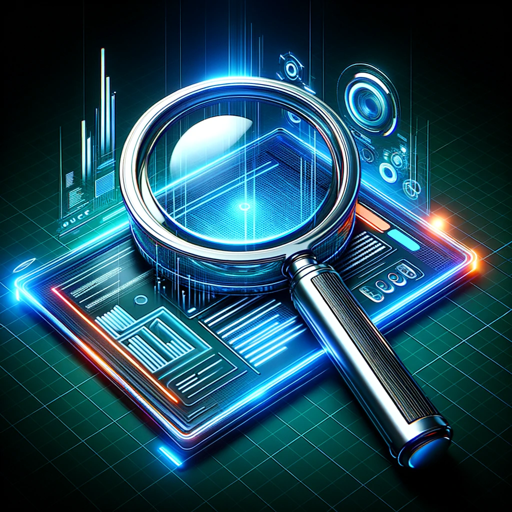
Apps Script
Automate Google Workspace tasks with AI.

Movie Poster Creator
AI-powered Pixar-style movie posters
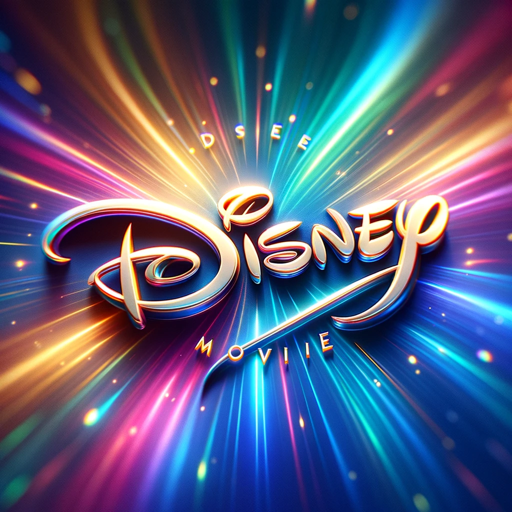
Invoice Bot
Effortlessly create professional invoices with AI.

2024 Market Outlooks Nerd
AI-powered insights for market outlooks.

Veterinarian GPT
AI-Powered Solutions for Pet Health
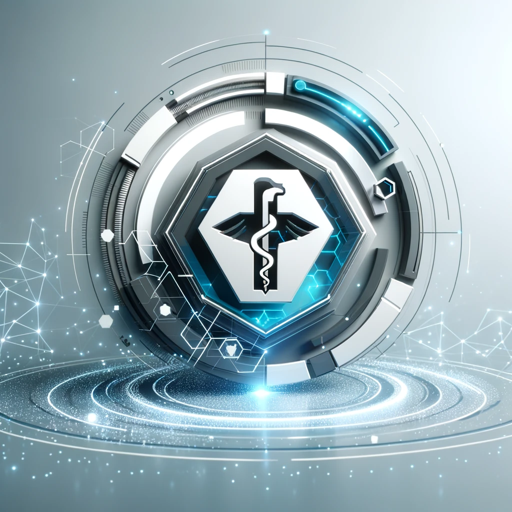
♣️ ClubGPT ♣️ - developer team in one
Your AI-powered development team in one tool.

论文文献总结
AI-powered literature summary and analysis.

NuxtBot
AI-powered assistant for Nuxt 3 development
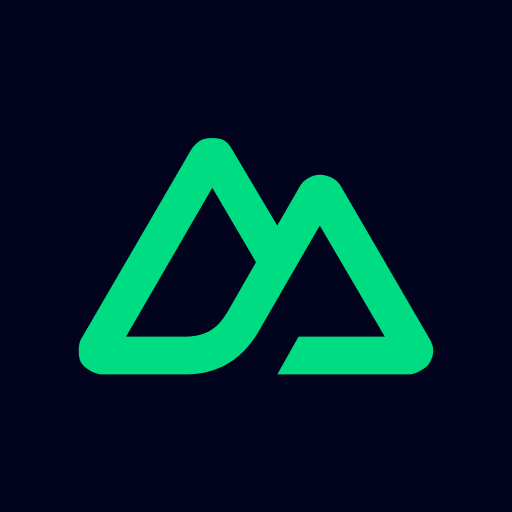
Buddha GPT - Buddhism Dhamma companion
AI-powered insights from early Buddhist texts.

Ecommerce SEO Product Description Listing Write AI
AI-Powered SEO for E-commerce Success

Marketing GPT
Your AI-powered marketing strategist.

- Performance Optimization
- Software Development
- System Programming
- Embedded Systems
- Data Structures
Common Questions About the C Programming Language
What is the C programming language?
C is a general-purpose, procedural programming language that supports structured programming. It was developed in the 1970s and has influenced many other programming languages.
How do I debug a C program?
Debugging C programs can be done using tools like GDB. By setting breakpoints and stepping through code, you can identify and fix issues in your program.
What are pointers in C?
Pointers in C are variables that store memory addresses. They are powerful but require careful handling to avoid memory leaks and segmentation faults.
What is the difference between `malloc` and `calloc`?
`malloc` allocates a block of memory without initializing it, while `calloc` allocates and initializes memory to zero.
How can I manage memory in C?
Memory management in C involves manually allocating and freeing memory using `malloc`, `calloc`, `realloc`, and `free`. Proper memory management is crucial to prevent leaks.