Kotlin-Kotlin development platform
AI-powered Kotlin development

Your personal Kotlin copilot, assistant, and project generator with a focus on responsive, beautiful, and scalable apps. Write clean code and become a much faster developer.
👤 Build a full login screen with password recovery
🪲 Find any bug or improvement in my code
⭐️ Transform this UI design into Kotlin code
💡 Teach me a useful skill or trick in Kotlin
Related Tools
Load More
Java
Your personal Java / Spring assistant and project generator with a focus on responsive and scalable code. Write clean code and become a much faster developer.
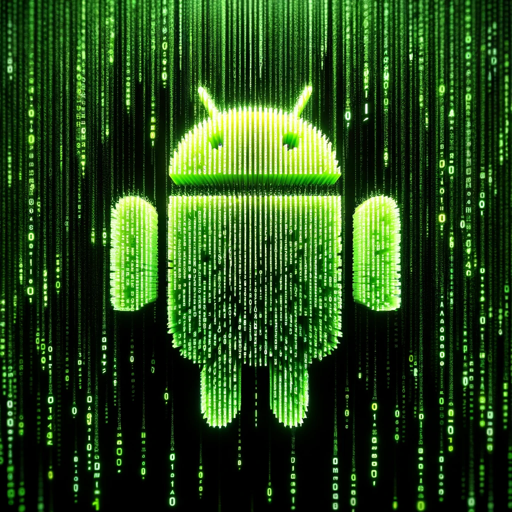
Android Studio GPT
GPT expert in Kotlin & Java.

Swift Developer
Swift Developer is an AI tailored for Apple family software engineering in Swift, offering solutions aligned with market best practices and swift.org guidelines. It provides clear, efficient code and simplifies complex concepts, ideal for optimizing and u

Kotlin Expert
Expert in Kotlin programming, offering tailored advice and solutions.

Android Studio Developer
⭐️ 4.4ㆍYour personal Kotlin, Jetpack Compose, and XML Layouts copilot and project generator, with a focus on responsive, beautiful, and scalable apps. Write clean code and become a much faster developer.
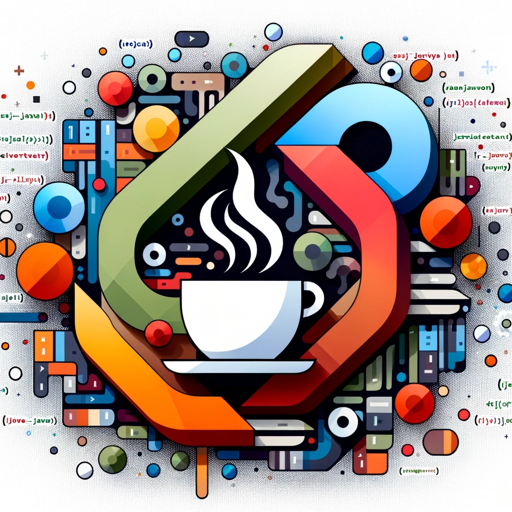
Java + Spring Boot Buddy
Helping developers with code review and generation. Adhering to best practices like SOLID, clean code and design patterns.
20.0 / 5 (200 votes)
Introduction to Kotlin
Kotlin is a statically-typed programming language that runs on the Java Virtual Machine (JVM) and can also be compiled to JavaScript or native code. It was developed by JetBrains and first released in 2011, with its first official stable release in 2016. Kotlin was designed to be a modern language that improves upon the limitations of Java while maintaining full interoperability with Java code. Its design purpose is to offer a more concise, expressive, and safer alternative to Java, making it easier to write and maintain large-scale applications. Kotlin has rapidly gained popularity, especially in Android development, due to its advanced features like null safety, extension functions, and higher-order functions. The language's versatility also makes it suitable for backend development, web development, and even data science scenarios.
Main Functions of Kotlin
Null Safety
Example
Kotlin's type system is designed to eliminate the danger of null references. By default, variables cannot hold null values unless explicitly declared nullable using the '?' syntax.
Scenario
In a large Android application, null safety helps prevent runtime crashes by ensuring that potential null values are handled at compile time. This leads to more stable and reliable apps.
Extension Functions
Example
Kotlin allows developers to extend a class with new functionality without having to inherit from the class or use design patterns like Decorator. For example, you can add a new method to a String class to check if it is a valid email address.
Scenario
In a web application, extension functions can be used to add utility methods to existing classes, making the code more readable and reusable. For instance, adding a 'isValidEmail()' function to the String class simplifies email validation across the codebase.
Coroutines
Example
Kotlin coroutines provide a way to write asynchronous, non-blocking code. They are a type of lightweight thread that allows for easier management of complex asynchronous operations.
Scenario
In an Android application, coroutines can be used to perform network requests on a background thread while keeping the UI thread responsive. For example, fetching data from an API without blocking the main thread ensures a smooth user experience.
Ideal Users of Kotlin
Android Developers
Android developers are one of the primary user groups for Kotlin. Google officially endorsed Kotlin as a first-class language for Android development in 2017. Kotlin's interoperability with Java, concise syntax, and powerful features like coroutines and extension functions make it ideal for building robust Android applications. Developers benefit from faster development times, reduced boilerplate code, and safer code due to features like null safety.
Backend Developers
Backend developers using JVM-based frameworks like Spring or Ktor can also greatly benefit from Kotlin. Its expressiveness and null safety lead to cleaner, more maintainable code, while full interoperability with Java allows developers to integrate Kotlin into existing Java-based systems easily. Kotlin's support for functional programming paradigms, along with features like data classes and type inference, helps backend developers write more concise and reliable code.
Guidelines for Using Kotlin
Visit aichatonline.org for a free trial without login
Begin by accessing aichatonline.org, which provides a free trial without requiring a login or a ChatGPT Plus subscription. This platform is essential for starting with Kotlin.
Install Kotlin and Set Up Your IDE
Download and install Kotlin by setting up an Integrated Development Environment (IDE) such as IntelliJ IDEA, Android Studio, or Visual Studio Code with Kotlin plugins.
Create a New Kotlin Project
Start a new project within your IDE. Choose Kotlin as your programming language, and set up the project structure, including dependencies, libraries, and configurations.
Write and Compile Kotlin Code
Write Kotlin code using the syntax and features of the language. Compile the code directly within the IDE to ensure there are no errors and that the program runs as expected.
Test and Deploy Your Kotlin Application
Test your Kotlin application thoroughly. Once confirmed, deploy the application on the desired platform, whether it's a mobile app, server-side application, or a desktop program.
Try other advanced and practical GPTs
Android Studio Developer
AI-Powered Assistant for Android Developers

Prompt Mestre 2.0
Enhance your AI outputs with precision.

Photo Realistic GPT
Transforming Text into Realistic Images with AI
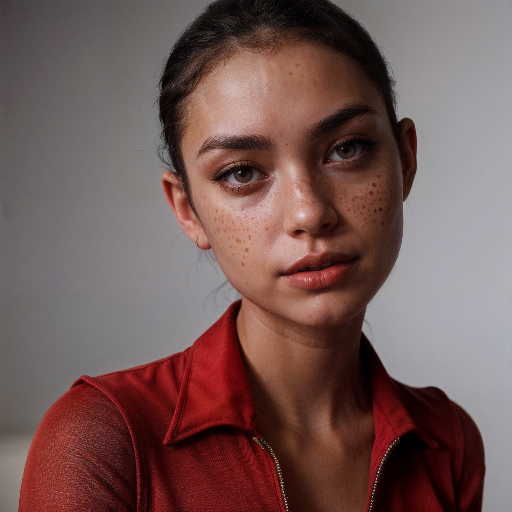
Library of Babel
Discover Books with AI-Powered Precision

Prompt Bug Buster
AI-powered precision in prompt crafting

Prompt Maker
Enhance your ideas with AI-driven precision.

Suno AI V3 - Lyrics
AI-driven lyrics creation for musicians

Rise AI - Investing Co-pilot
AI-Driven Investment and Wealth Guidance

C++ (Cpp)
AI-powered C++ coding assistant.

中文paraphrase
AI-powered Chinese text paraphrasing
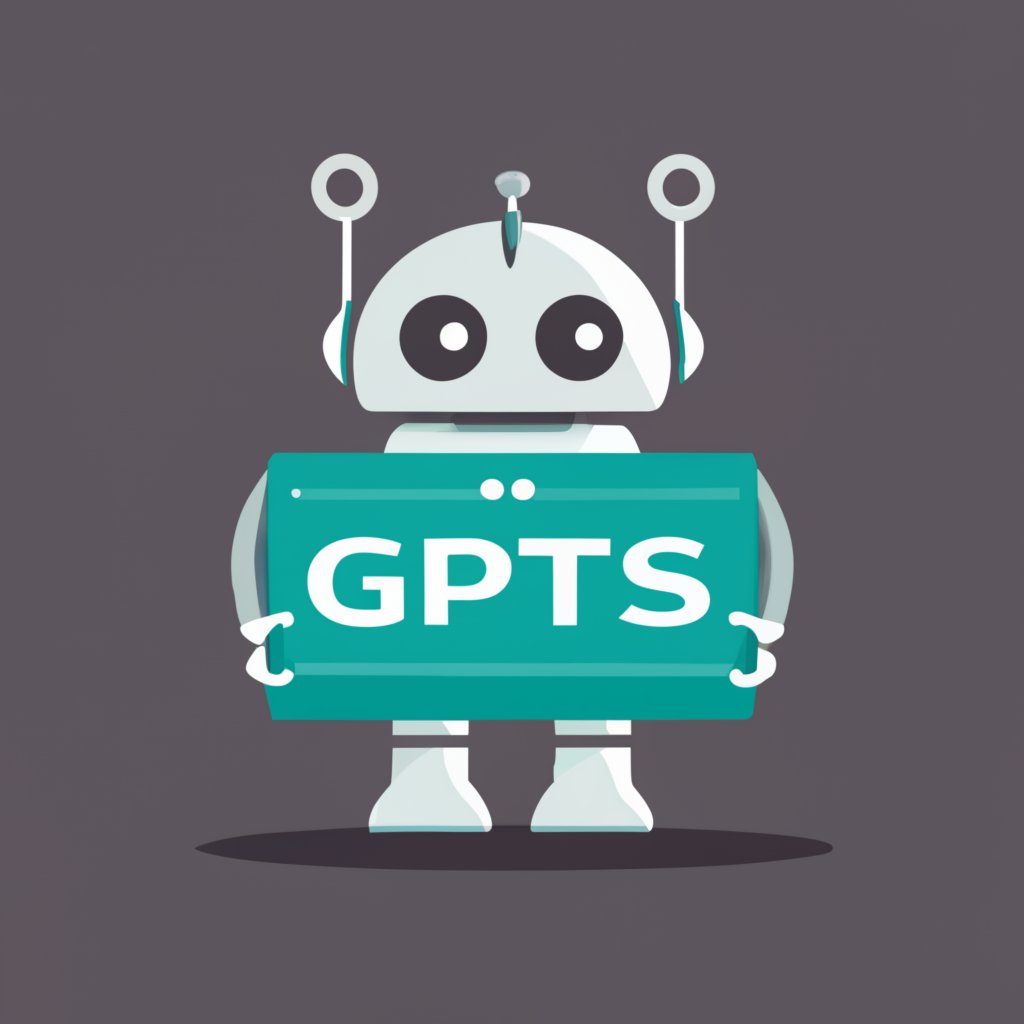
Law: Legal & Contract (Not Human Lawyer)
AI-powered legal document assistant.

ㆍYouTube ⚡️ Fast Summaryㆍ
AI-powered summaries for YouTube videos.

- Cross-Platform
- Mobile Development
- Null Safety
- Server-Side
- Asynchronous
Common Questions About Kotlin
What is Kotlin, and why should I use it?
Kotlin is a statically-typed programming language developed by JetBrains. It is fully interoperable with Java, making it an excellent choice for Android development, backend services, and cross-platform applications. Its concise syntax, modern features, and strong community support are key reasons to use it.
How does Kotlin handle null safety?
Kotlin natively handles null safety by distinguishing between nullable and non-nullable data types. This feature helps in reducing the occurrence of NullPointerExceptions, making your code more robust and less error-prone.
Can Kotlin be used for server-side development?
Yes, Kotlin is widely used for server-side development. It works well with popular frameworks like Spring Boot and Ktor, offering a modern and efficient environment for building web applications and microservices.
Is Kotlin suitable for cross-platform development?
Absolutely. Kotlin's multiplatform capabilities allow developers to share code between Android, iOS, and the web. This makes it an effective tool for building cross-platform applications, ensuring consistency and reducing development time.
What are Kotlin Coroutines, and why are they important?
Kotlin Coroutines are a powerful tool for asynchronous programming. They simplify writing concurrent code by providing a lightweight and more readable alternative to threads, enhancing performance and efficiency in applications.