Rust-Rust guide and tools
AI-Powered Rust Programming Assistant

Powerful Rust coding assistant. Trained on a vast array of the best up-to-date Rust resources, libraries and frameworks. Start with a quest! 🥷 (V1.9)
Ask Samurai 🥷
Optimize this Rust function:
Tutorial 📚
Convert this C++ to Rust:
Related Tools
Load More
R Wizard
A specialist in R programming, skilled in Data Science, Statistics, and Finance, providing accurate and useful guidance.

Rust
Your personal Rust assistant and project generator with a focus on responsive, efficient, and scalable code. Write clean code and become a much faster developer.

Rblox LUA Programming GOD
This will help you with Roblox Studio, Lua, Placement of Scripts, Navigate Menus, Objects and more.

Ruby on Rails
Your personal Ruby on Rails assistant and code generator with a focus on responsive, efficient, and scalable projects. Write clean code and become a much faster developer.

Ruby On Rails
Ruby on Rails Mentor
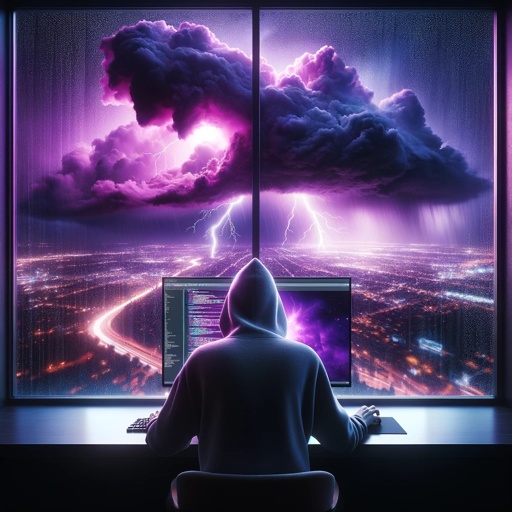
h4ckGPT
Your personal security tool
20.0 / 5 (200 votes)
Introduction to Rust
Rust is a modern systems programming language that focuses on safety, speed, and concurrency. It is designed to provide the low-level control and performance of languages like C and C++, but with a strong emphasis on preventing common programming errors such as null pointer dereferences, buffer overflows, and data races. Rust achieves memory safety without a garbage collector, instead using a strict ownership model and a system of borrowing and lifetimes to ensure references are always valid. This makes Rust especially suitable for building safe and efficient software, including operating systems, game engines, and web servers. For example, Rust's ownership system prevents data races at compile time, which is a common issue in concurrent programming. In practice, this means that once a Rust program compiles, it is often free from certain classes of runtime errors, giving developers more confidence in the correctness of their code. Additionally, Rust has a strong and growing ecosystem, supported by the Cargo package manager, which simplifies dependency management and builds processes for developers. This has made Rust increasingly popular for both new projects and for rewriting critical components of existing systems to improve safety and performance【12:2†source】【12:19†source】.
Main Functions of Rust
Memory Safety
Example
Using Rust’s ownership system, a developer can create a vector of integers and safely pass it between threads without risking data races.
Scenario
Consider a real-time data processing application that handles large volumes of data concurrently. Rust's memory safety guarantees allow developers to process data across multiple threads efficiently without worrying about common concurrency issues like race conditions. This is particularly important in high-performance computing environments where data integrity and application stability are critical【12:0†source】【12:3†source】.
Zero-cost Abstractions
Example
Implementing iterators in Rust that are as efficient as manually written loops in C, thanks to Rust's powerful inlining and optimization capabilities.
Scenario
In game development, where performance is key, Rust allows developers to use high-level abstractions like iterators and closures without incurring a runtime performance penalty. This makes it easier to write clean, maintainable code while still meeting the performance demands of modern games【12:19†source】【12:11†source】.
Concurrency
Example
Using Rust’s concurrency primitives like `std::thread` and `std::sync` to manage threads and shared state safely.
Scenario
Building a web server that handles thousands of concurrent connections efficiently is a common use case for Rust. By leveraging Rust's concurrency features, developers can ensure that their server is both fast and robust, capable of handling many connections simultaneously without crashing due to race conditions or memory errors【12:14†source】【12:10†source】.
Ideal Users of Rust
Systems Programmers
Systems programmers who are looking to build operating systems, embedded systems, or other low-level software where control over hardware and performance is crucial will benefit from Rust. The language's focus on safety and performance without a garbage collector makes it ideal for these environments. Additionally, Rust's ability to produce highly optimized binaries means that developers can create software that runs efficiently on resource-constrained devices【12:18†source】【12:9†source】.
Web Developers
Web developers working on server-side applications, such as high-performance web servers or backend services, can leverage Rust's safety and concurrency features to build robust, scalable systems. Rust's growing ecosystem, including frameworks like Actix and Rocket, provides developers with the tools they need to create efficient web applications. Rust's emphasis on security and correctness helps prevent vulnerabilities that could be exploited in production environments【12:13†source】【12:5†source】.
How to Use Rust
Visit aichatonline.org for a free trial without login, also no need for ChatGPT Plus.
This platform offers an accessible trial experience, where you can start exploring Rust without any upfront commitments.
Install Rust and Cargo
Use the command `curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh` to install Rust and its package manager, Cargo. Follow the on-screen instructions to complete the setup.
Set Up Your Development Environment
Use an IDE like Visual Studio Code or JetBrains CLion with the Rust plugin for syntax highlighting, code completion, and integrated debugging. This will make development more efficient.
Learn the Basics
Start with resources like 'The Rust Programming Language' (The Book) and 'Rust By Example' to understand Rust's syntax, ownership model, and basic constructs.
Build and Run Your First Project
Create a new project with `cargo new project_name`, navigate into the directory, and run `cargo build` to compile and `cargo run` to execute your program. Experiment with code to reinforce your learning.
Try other advanced and practical GPTs
Haskell GPT
Enhance your Haskell coding with AI-driven insights.

GA4 SQL
AI-powered GA4 SQL query builder.

Numerology Sage
AI-Powered Numerology for Self-Discovery
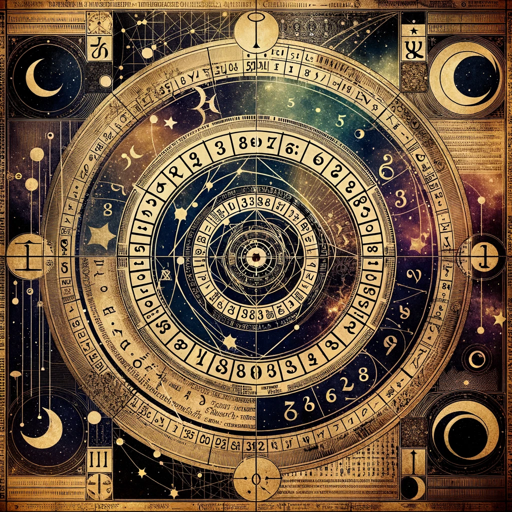
Interview Wizard GPT
Your AI-Powered Guide to Interview Success

GPT-Builders' Assistant
AI-powered assistant for custom GPT building.

DnD GPT
AI-Powered Dungeon Master for Immersive Play.

X Optimizer GPT
AI-powered content optimization for maximum engagement.
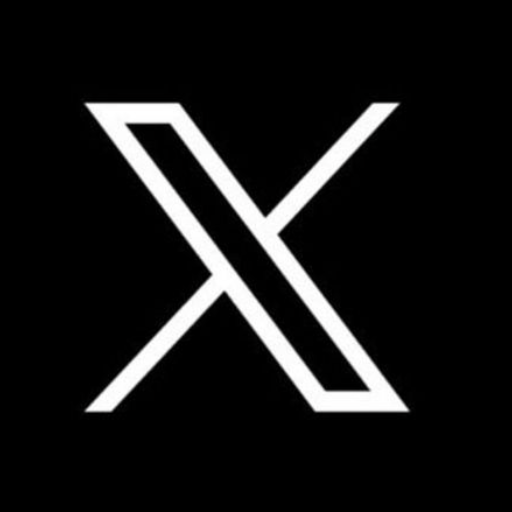
Shopping Scout
AI-Powered Shopping Companion for Amazon.
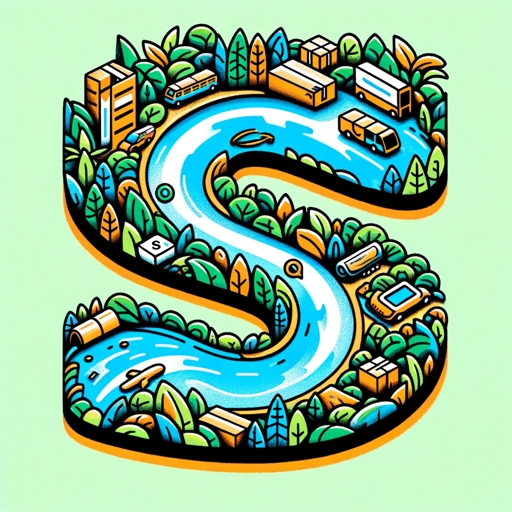
GPT Creator
AI-powered GPT creation, simplified.
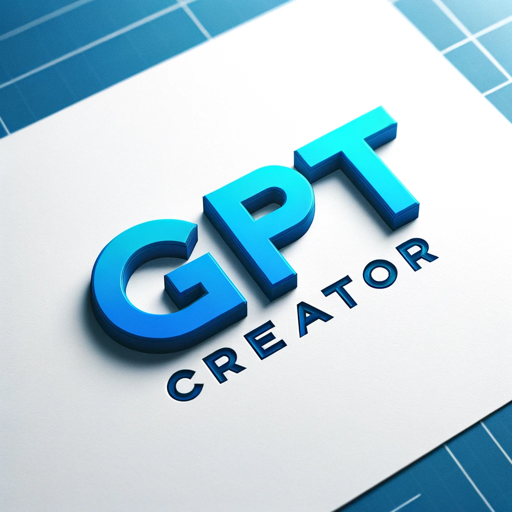
NGINX Guru
AI-driven NGINX configuration and support.
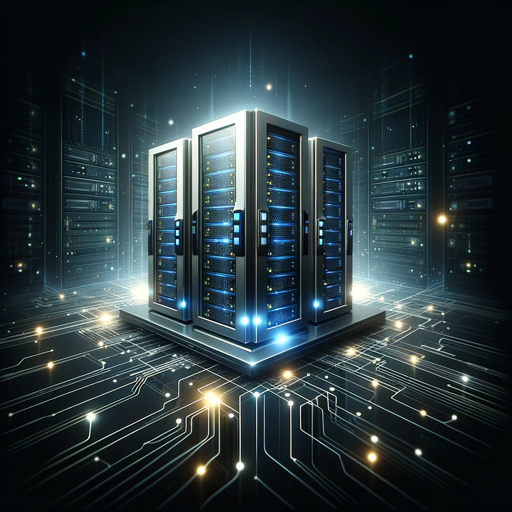
SPARK ✧
AI-powered creative tool for personalized content.

Buyer Persona Generator
AI-Powered Buyer Persona Generation

- Web Development
- Game Development
- System Programming
- Embedded Systems
- Concurrency
Rust Q&A
What is Rust used for?
Rust is used for system-level programming, web development, game development, and concurrent applications, among other things. Its memory safety guarantees make it ideal for performance-critical software.
How does Rust ensure memory safety?
Rust uses a unique ownership model, enforced at compile time, to ensure memory safety without needing a garbage collector. It prevents data races and null pointer dereferencing.
What are some common use cases for Rust?
Rust is commonly used for developing operating systems, web servers, network services, and command-line tools. It's also favored in scenarios where performance and reliability are critical.
How does Rust's borrowing system work?
Rust's borrowing system allows you to have multiple references to data, either mutable or immutable, but never both at the same time. This ensures safe concurrency and prevents data races.
Why is Rust popular in the software development community?
Rust is popular because it offers the performance of C/C++ with safer memory management, reducing bugs and improving code reliability. Its modern features and vibrant community also contribute to its popularity.